Dynamic Travel widget: configuration
Here you can find details to how correctly configure the dynamic travel widget (dynamic installments feature will be displayed).
When should I use the dynamic travel widget?
You should install the Scalapay dynamic travel widget when your agrement with the Scalapay Sales referent includes product dynamicity (pay in 3 or pay in 4) according to determined parameters, that are travelDate and/or price of the reservation.
How easy it is to render the travel version of Scalapay widget?
Simply install the widget scripts, set the channel attribute to travel and, follwing the instructions below, configure the correct attributes relative to the travel date.
Since the installments are dynamic the number-of-installments and frequency-number attributes can be omitted.
1. Include Scalapay scripts
Add Scalapay scripts below into head tag of product or cart HTML page:
<script type="module" src="https://cdn.scalapay.com/widget/v4/js/scalapay-widget.esm.js"></script>
<script nomodule src="https://cdn.scalapay.com/widget/v4/js/scalapay-widget.js"></script>
If you already have the default widget (v3), you must remove the old scripts before adding the new ones (v4)
2. Add scalapay HTML tag in the page template
Place the widget in the desired location in the HTML of the product, cart and checkout pages
Settings
Attribute | Suggested value | Description |
---|---|---|
channel | travel | The widget channel. Must be equal to travel to render the widget travel version |
threshold-days | 45 (optional) | Value, along with threshold-price, after which pay in 4 will be rendered (instead of pay in 3) |
threshold-price | threshold-price | threshold-price |
date | Must be in the yyyy-MM-dd ISO format (e.g. 2023-09-30) | Date of travel departure used for the dynamic installments calculation. The date attribute of the widget must be updated by you each time the travel date changes in the frontend |
N.B.
If threshold-days
and threshold-price
are not set, the default values are used (45 days and € 999.99).
Example of widget with dynamic rule equals to : if amount >= € 1500 AND date - now >= 30 days ⇒ render pay in 4, else pay in 3
.
<scalapay-widget
type='product'
channel='travel'
hide='false'
hide-price='false'
min='5'
max='2500'
amount-selectors='["#price-container"]'
currency-position='before'
currency-display='symbol'
logo-size='100'
theme='primary'
locale='en'
date-selectors='["#travel-date-container .show-date"]'
date-format="(?<day>\d\d)\s(?<month>\w{3}$)"
threshold-days='30'
threshold-price='1500'
></scalapay-widget>
Date attribute
The date attribute of the widget must be updated by you each time the travel date changes in the frontend.
// example of custom implementation.
document.addEventListener('DOMContentLoaded', () => {
const observer = new MutationObserver(() => {
const newDate = document.querySelector('#travel-date-element')?.textContent;
const newDateISO = newDate.myParseFx('yyyy-MM-dd');
const scalapayWidget = document.querySelector('.container scalapay-widget');
scalapayWidget.setAttribute('date', newDateISO);
});
observer.observe(document.querySelector("#travel-date-element"),
{subtree: true, childList: true, attributes: true});
});
});
Please follow the instrucitons below in case date parameter must me retreived differently from what described above
Attribute | Suggested value | Description |
---|---|---|
date-selectors | e.g. [".container #travel-date-value"] | List of selectors for the departure travel date. Based on the HTML of the page where the widget is rendered, set the travel date selectors here. |
date-format | Regex defined with 2-3 capture groups as specified below | Regex used to retrieve the travel date from the selectors list. |
(?<day>{your_day_regex_here}){your_separator_regex_here} \
(?<month>{your_month_regex_here}){your_separator_regex_here} \
(?<year>{your_year_regex_here})
- The regex MUST contain 2 named captured groups (
day
,month
) plus 1 optional (year
). If the year capture group is missing the current year will be used - The regex must be built considering the clean date format, possible HTML tags in between or around will be removed before the validation
Example HTML | Example Regex (date-format attribute) |
---|---|
Departure: <b>30</b> Jun - Way back: <b>15</b> Jul | (?<day>\\d\\d)\\s(?<month>\\w{3}) |
<div id="travel-date-container"> <meta content="xyz"/>06.08.2023 </div> | (?<day>\\d\\d)\.(?<month>\\d\\d)\.(?<year>\\d\\d\\d\\d$) |
<div class="date-periods"> <i class="calendar-icon"></i> From 24/07/2023 - <i class="calendar-icon"></i> to 30/07/2023 (6 nights) </div> | (?<day>\\d\\d)/(?<month>\\d\\d)/(?<year>\\d{4}) |
<div id="travel-date-container"> <span><em>01-09-2023</em> <br/>19:35 </span> </div> | (?<day>\\d\\d)-(?<month>\\d\\d)-(?<year>\\d{4}) |
Example
<!doctype html>
<html>
<head>
<title>Scalapay Widget Dynamic Travel Product Page</title>
<script type="module" src="https://cdn.scalapay.com/widget/scalapay-widget-loader.js"></script>
</head>
<body>
<div id="price-container">€ 2100.00</div>
<div id="travel-date-container">
<span class="show-date">14 Jul</span>
</div>
<scalapay-widget
type='product'
channel='travel'
hide='false'
hide-price='false'
min='5'
max='3000'
amount-selectors='["#price-container"]'
currency-position='before'
currency-display='symbol'
logo-size='100'
theme='primary'
locale='en'
date-selectors='["#travel-date-container .show-date"]'
date-format="(?<day>\d\d)\s(?<month>\w{3}$)"
></scalapay-widget>
</body>
</html>
<!doctype html>
<html>
<head>
<title>Scalapay Widget Travel Checkout Page</title>
<script type="module" src="https://cdn.scalapay.com/widget/scalapay-widget-loader.js"></script>
</head>
<body>
<div id="travel-date-container">
<p>Your Departure: </p>
<span class="show-date">14/07/2023</span>
</div>
<div id="price-container">€ 2100.00</div>
<scalapay-widget
type='checkout'
channel='travel'
show-title='true'
hide='false'
hide-price='false'
min='5'
max='3000'
amount-selectors='["#price-container"]'
currency-position='before'
currency-display='symbol'
logo-size='100'
theme='primary'
locale='en'
date-selectors='["#travel-date-container .show-date"]'
date-format="(?<day>\d\d)/(?<month>\d\d)/(?<year>\d\d\d\d$)"
></scalapay-widget>
</body>
</html>
Dynamic Travel widget product and cart page - Full example
<!doctype html>
<html>
<head>
<title>Scalapay Widget Dynamic Travel Product Page</title>
<script type="module" src="https://cdn.scalapay.com/widget/scalapay-widget-loader.js"></script>
</head>
<body>
<div id="price-container">€ 2100.00</div>
<div id="travel-date-container">
<span class="show-date">14 Jul</span>
</div>
<scalapay-widget
type='product'
channel='travel'
hide='false'
hide-price='false'
min='5'
max='3000'
amount-selectors='["#price-container"]'
currency-position='before'
currency-display='symbol'
logo-size='100'
theme='primary'
locale='en'
date="2023-07-14"
></scalapay-widget>
</body>
</html>
Please note
The type and channel attributes must be set according to the example above
The min and max attributes pertain to the contractual terms agreed with Scalapay, please check the contract to configure them (then refer to the above example)
All the other parameters must be configured according to your website characteristics and preferences
Dynamic Travel widget checkout - Full example
<!doctype html>
<html>
<head>
<title>Scalapay Widget Travel Checkout Page</title>
<script type="module" src="https://cdn.scalapay.com/widget/scalapay-widget-loader.js"></script>
</head>
<body>
<div id="travel-date-container">
<span class="show-date">Departure: 14 Jul 2023</span>
</div>
<div id="price-container">€ 2100.00</div>
<scalapay-widget
type='checkout'
channel='travel'
show-title='true'
hide='false'
hide-price='false'
min='5'
max='3000'
amount-selectors='["#price-container"]'
currency-position='before'
currency-display='symbol'
logo-size='100'
theme='primary'
locale='en'
date="2023-07-14"
></scalapay-widget>
</body>
</html>
Please note
The channel and type attributes must be set according to the example above
The min and max attributes pertain to the contractual terms agreed with Scalapay, please check the contract to configure them (then refer to the above example)
All the other parameters must be configured according to your website characteristics and preferences
Best practices
Product page/Listing page
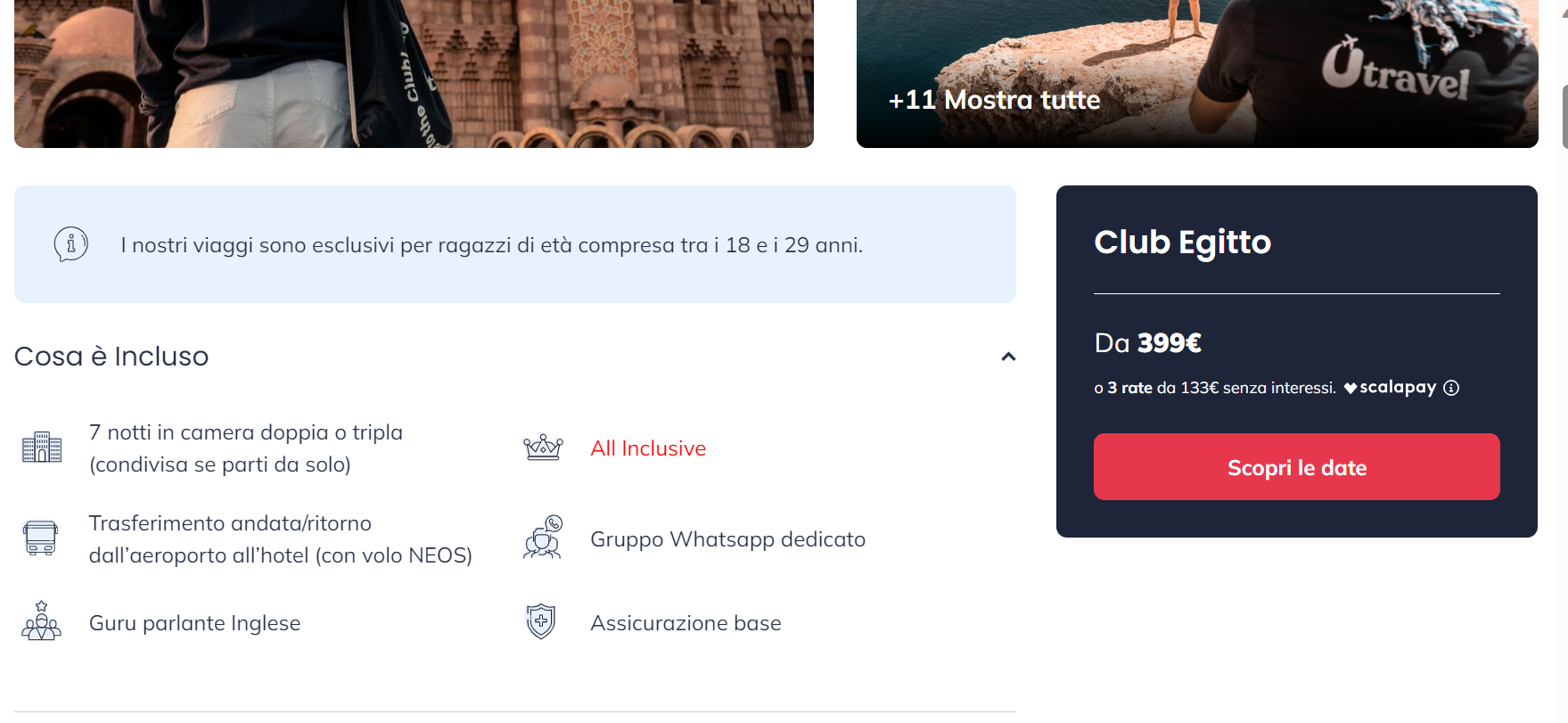
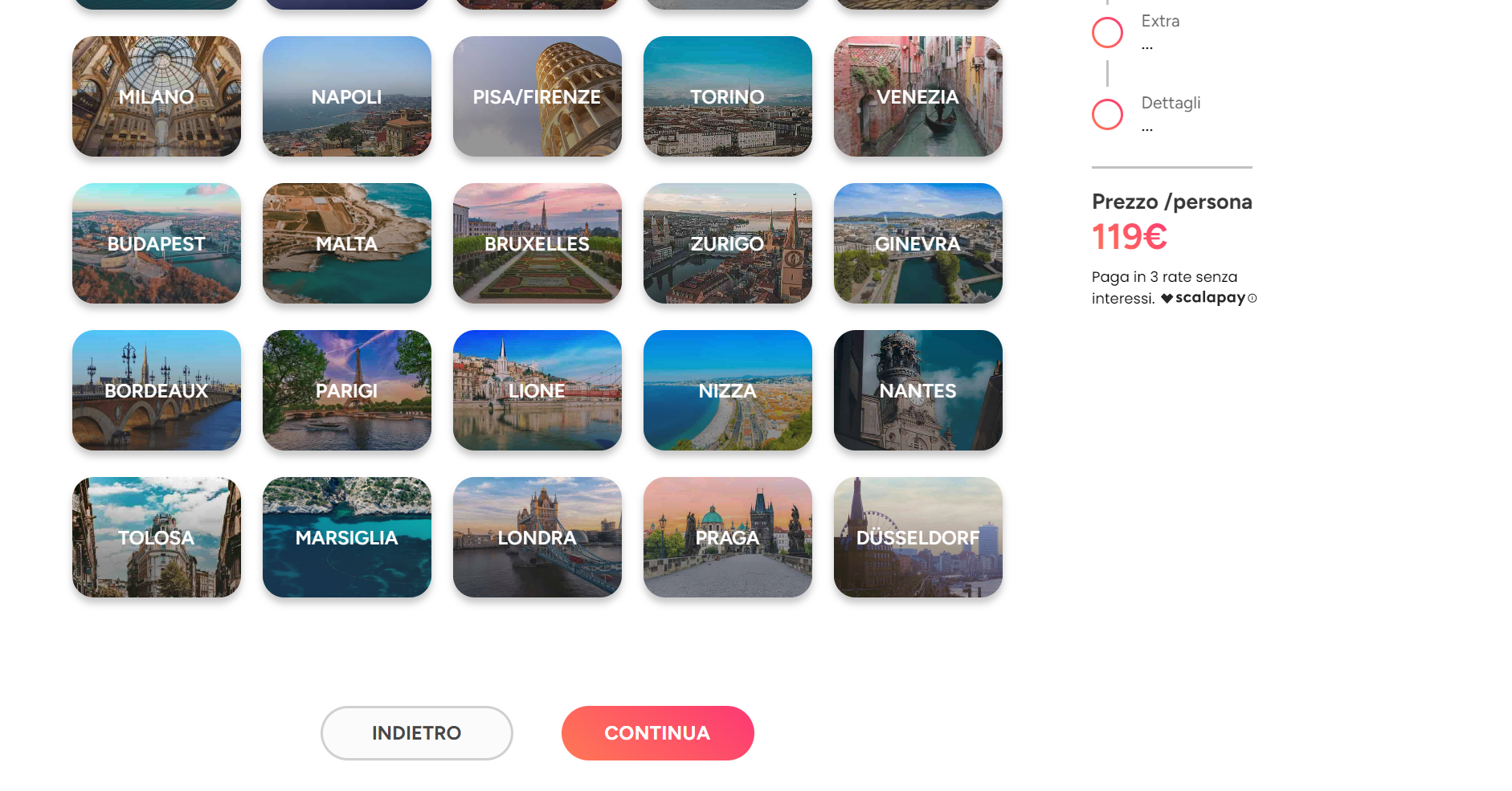
Cart page/Recap page
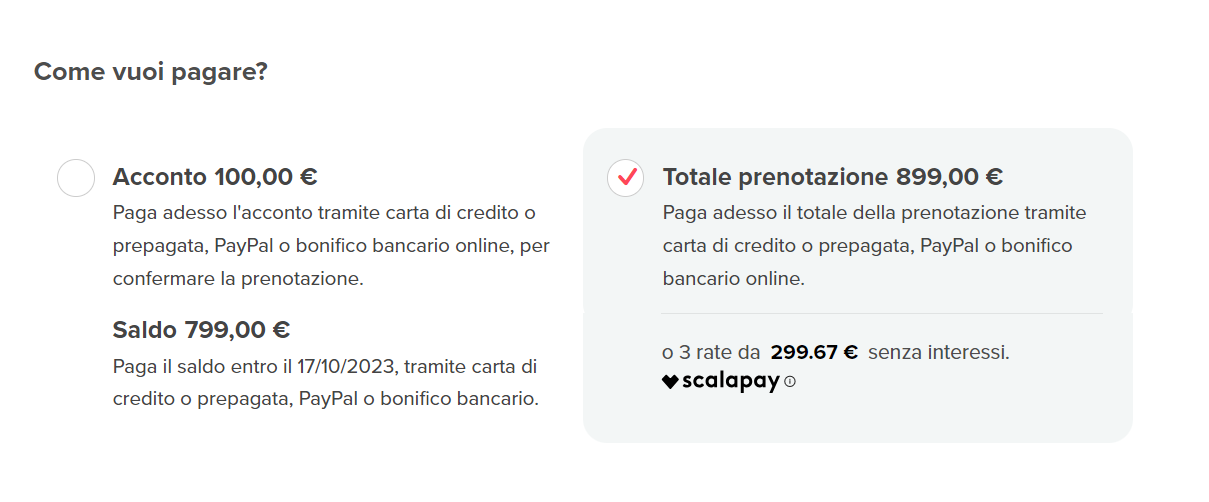
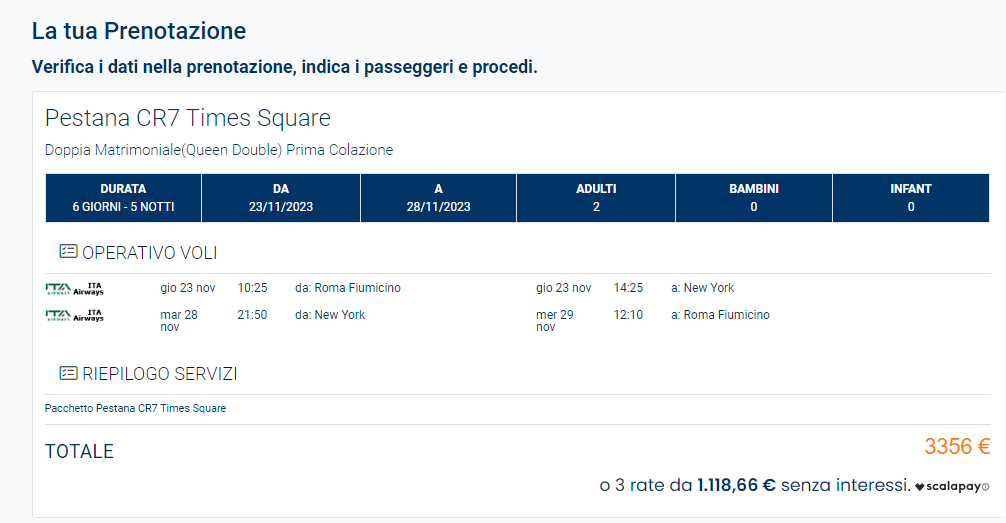
Checkout page
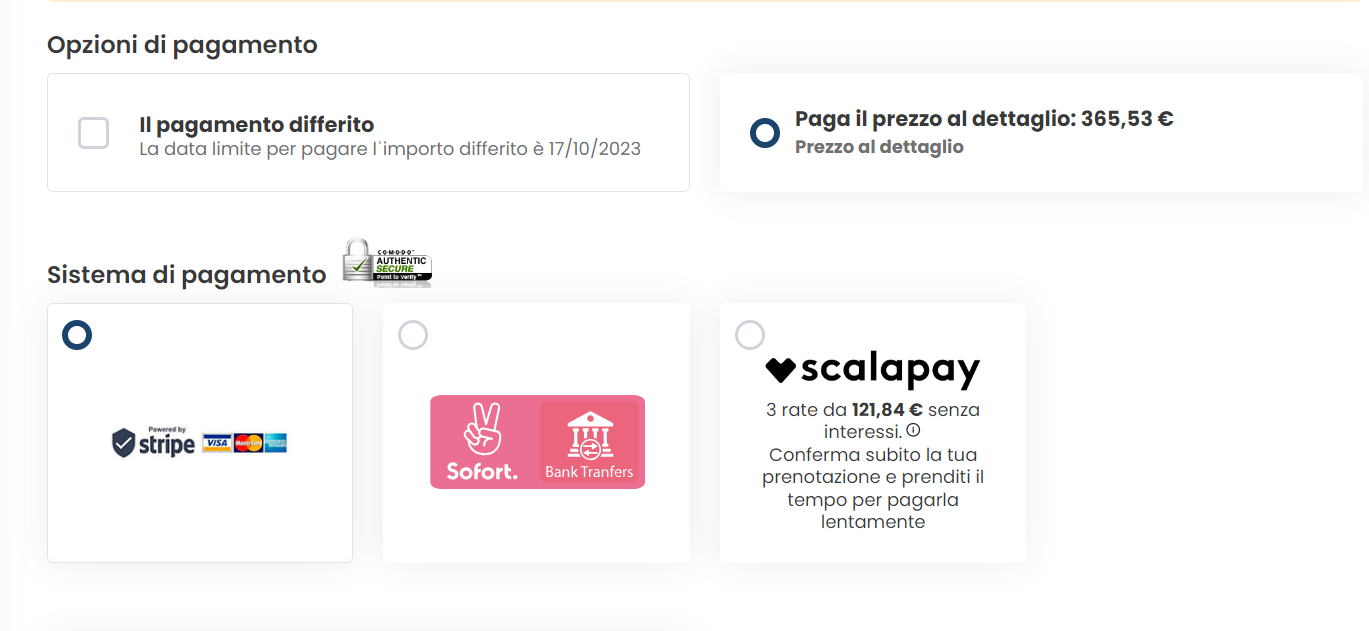
Updated 2 months ago